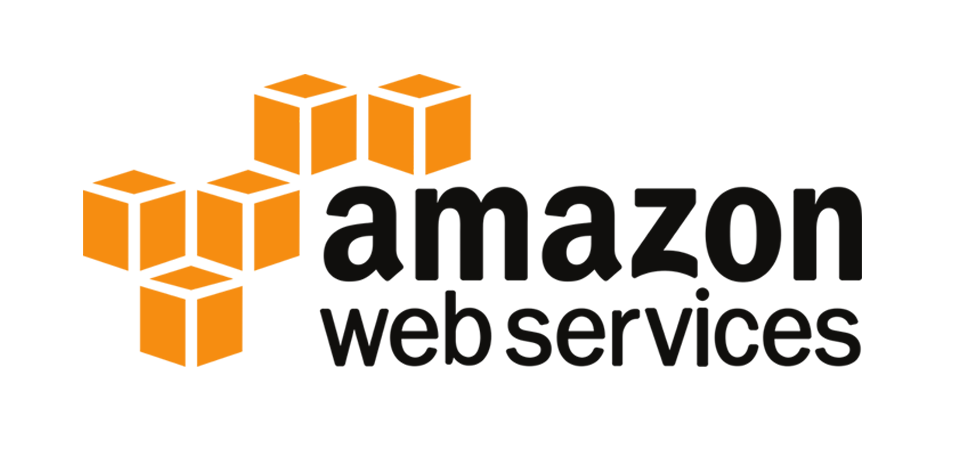
When creating an HTTP/HTTPS subscription in AWS SNS, you can observe that the subscription is hanging in the status: “Pending confirmation”

SNS to the specified URL makes a POST request in which it sends data in JSON format and expects to receive a key value in response: “SubscribeURL“. But if the application does not know how to respond to SNS, then you can enter the confirmation URL manually, but for this you need to find out.
To do this, you can use Nginx and its access_log.
Since the body of the POST request is written to the log only when using “proxy_pass“, we model proxing to the backend.
nginx.conf
user nginx;
worker_processes 1;
error_log /var/log/nginx/error.log warn;
pid /var/run/nginx.pid;
events {
worker_connections 1024;
}
http {
log_format postdata escape=json '"$request_body"';
server {
listen 80;
server_name _;
location /success {
return 200;
}
location / {
proxy_redirect off;
proxy_pass_request_body on;
proxy_pass $scheme://127.0.0.1:$server_port/success;
add_header X-Body $request_body;
access_log /var/log/nginx/post.log postdata;
}
}
}
In the example, the configuration is for the HTTP protocol, if you have HTTPS, add the parts of the configuration that are related to SSL.
Reload the Nginx configuration:
systemctl reload nginx
After we return to the AWS console and select the necessary one in SNS subscriptions and make a one more request “Request Confirmation”
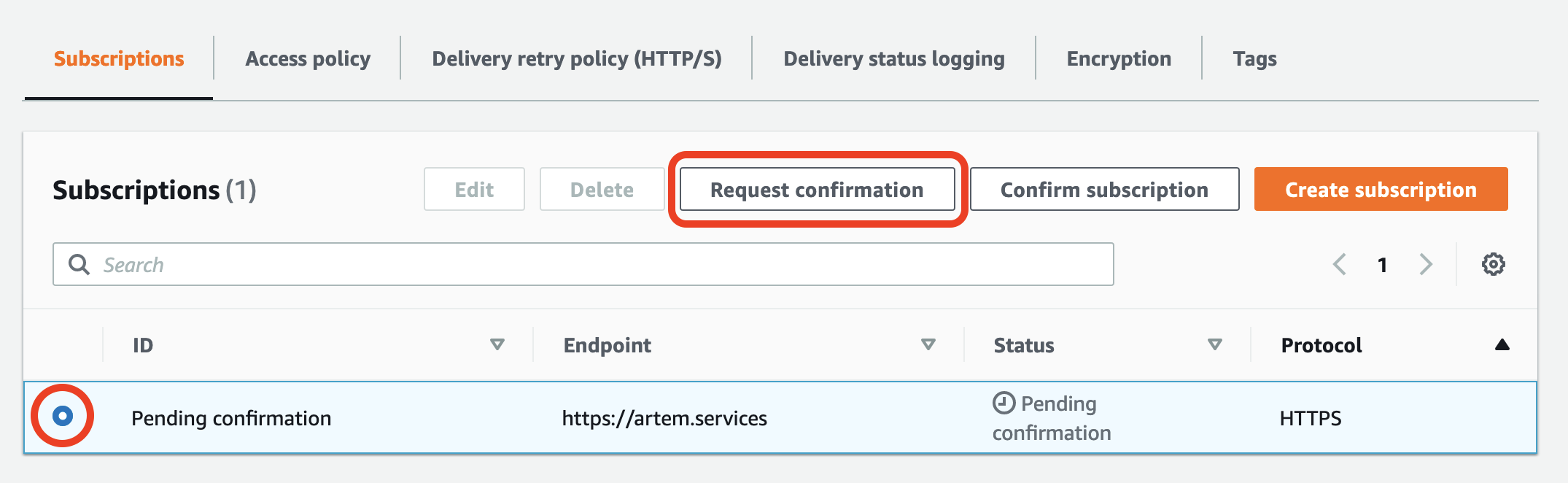
Follow to Nginx log:
cat /var/log/nginx/post.log
Interested in the value of the key: “SubscribeURL“, it will be of the form:
https://sns.{REGION}.amazonaws.com/?Action=ConfirmSubscription&TopicArn=arn:aws:sns:{REGION}:{YOUR_ACCOUNT_ID}:{YOUR_TOPIC_NAME}&Token={YOUR_TOKEN}
We copy it and return to AWS SNS. Select “Confirm Subscription”
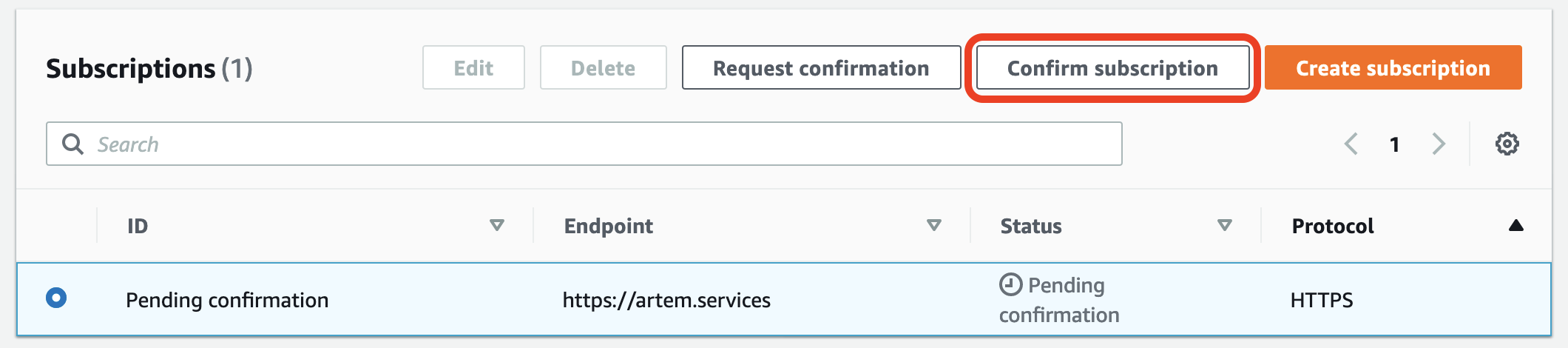
Insert the value “SubscribeURL” and confirm.
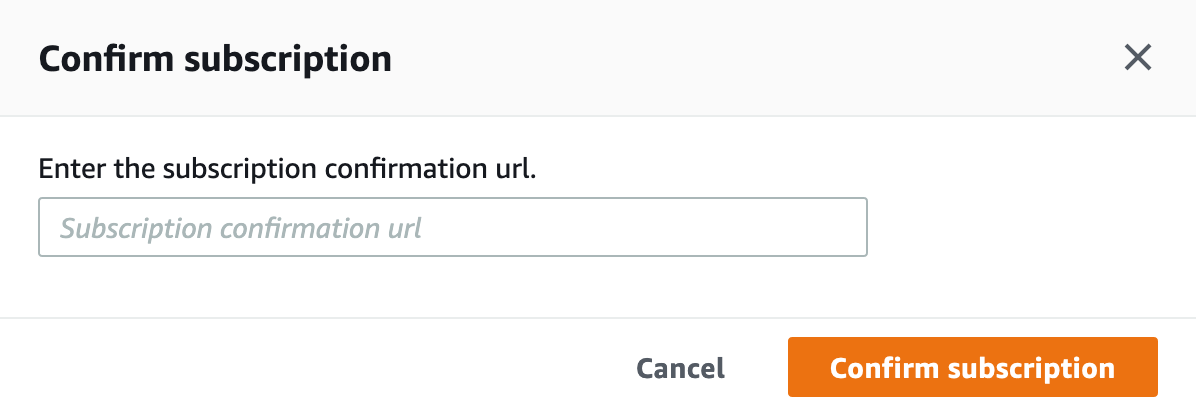